特征工程是將原始數(shù)據(jù)轉(zhuǎn)換為有意義的特征,以供機(jī)器學(xué)習(xí)算法使用并進(jìn)行準(zhǔn)確預(yù)測(cè)的過程。它涉及選擇、提取和轉(zhuǎn)換特征,以增強(qiáng)模型的性能。良好的特征工程可以極大地提高模型的準(zhǔn)確性,而糟糕的特征工程則可能導(dǎo)致性能不佳。
Fig.1 — Feature Engineering
在本指南中,我們將介紹一系列常用的特征工程技術(shù)。我們將從特征選擇和提取開始,這涉及識(shí)別數(shù)據(jù)中最重要的特征。然后,我們將轉(zhuǎn)向編碼分類變量,這是處理非數(shù)字?jǐn)?shù)據(jù)時(shí)的重要步驟。我們還將涵蓋縮放和歸一化、創(chuàng)建新特征、處理不平衡數(shù)據(jù)、處理偏斜和峰度、處理稀有類別、處理時(shí)間序列數(shù)據(jù)、特征變換、獨(dú)熱編碼、計(jì)數(shù)和頻率編碼、分箱、分組和文本預(yù)處理等內(nèi)容。
通過本指南,您將全面了解特征工程技術(shù)及其如何用于提高機(jī)器學(xué)習(xí)模型的性能。讓我們開始吧!
目錄
1.特征選擇和提取
2.編碼分類變量
3.縮放和歸一化
4.創(chuàng)建新特征
5.處理不平衡數(shù)據(jù)
6.處理偏斜和峰度
7.處理稀有類別
8.處理時(shí)間序列數(shù)據(jù)
9.文本預(yù)處理
特征選擇和提取
特征選擇和提取是機(jī)器學(xué)習(xí)中必不可少的部分,它涉及從數(shù)據(jù)集中選擇最相關(guān)的特征,以提高模型的準(zhǔn)確性和效率。在這里,我們將討論一些流行的特征選擇和提取方法,并提供 Python 代碼片段。
** 1.主成分分析(PCA)** :PCA 是一種降維技術(shù),通過找到一個(gè)能夠捕獲數(shù)據(jù)中最大方差的新特征集,從而減少數(shù)據(jù)集中的特征數(shù)量。新特征稱為主成分,它們彼此正交并可用于重構(gòu)原始數(shù)據(jù)集。
讓我們看看如何使用 scikit-learn 對(duì)數(shù)據(jù)集執(zhí)行 PCA:
from sklearn.decomposition import PCA
# create a PCA object
pca = PCA(n_components=2)
# fit and transform the data
X_pca = pca.fit_transform(X)
# calculate the explained variance ratio
print("Explained variance ratio:", pca.explained_variance_ratio_)
在這里,我們創(chuàng)建一個(gè) PCA 對(duì)象并指定要提取的主成分?jǐn)?shù)量。然后,我們擬合和轉(zhuǎn)換數(shù)據(jù)以獲得新的特征集。最后,我們計(jì)算解釋的方差比率以確定每個(gè)主成分捕獲了多少數(shù)據(jù)中的方差。
** 2.線性判別分析(LDA)** :LDA 是一種用于分類問題中的特征提取的監(jiān)督學(xué)習(xí)技術(shù)。它通過找到一個(gè)新的特征集,最大化數(shù)據(jù)中類別之間的分離程度。
讓我們看看如何使用 scikit-learn 在數(shù)據(jù)集上執(zhí)行 LDA:
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
# create an LDA object
lda = LinearDiscriminantAnalysis(n_components=1)
# fit and transform the data
X_lda = lda.fit_transform(X, y)
在這里,我們創(chuàng)建一個(gè) LDA 對(duì)象并指定要提取的主成分?jǐn)?shù)量。然后,我們擬合和轉(zhuǎn)換數(shù)據(jù)以獲得新的特征集。
3.相關(guān)分析 :相關(guān)分析用于識(shí)別數(shù)據(jù)集中特征之間的相關(guān)性。高度相關(guān)的特征可以從數(shù)據(jù)集中刪除,因?yàn)樗鼈兲峁┝巳哂?a target="_blank">信息。
讓我們看看如何使用 pandas 在數(shù)據(jù)集上執(zhí)行相關(guān)分析:
import pandas as pd
# calculate the correlation matrix
corr_matrix = df.corr()
# select highly correlated features
high_corr = corr_matrix[abs(corr_matrix) > 0.8]
# drop highly correlated features
df = df.drop(high_corr.columns, axis=1)
在這里,我們使用 pandas 計(jì)算相關(guān)矩陣并選擇高度相關(guān)的特征。然后,我們使用 drop 方法從數(shù)據(jù)集中刪除高度相關(guān)的特征。
Fig.2 — Feature Selection Measures
** 4. 遞歸特征消除(RFE)** :RFE 是一種通過逐步考慮越來越小的特征子集來選擇特征的方法。在每次迭代中,模型使用剩余的特征進(jìn)行訓(xùn)練,并對(duì)每個(gè)特征的重要性進(jìn)行排名。然后消除最不重要的特征,并重復(fù)該過程,直到獲得所需數(shù)量的特征為止。
以下是使用 RFE 進(jìn)行特征選擇的示例:
from sklearn.feature_selection import RFE
from sklearn.linear_model import LinearRegression
from sklearn.datasets import load_boston
data = load_boston()
X, y = data.data, data.target
model = LinearRegression()
rfe = RFE(model, n_features_to_select=5)
rfe.fit(X, y)
selected_features = data.feature_names[rfe.support_]
print(selected_features)
** 5.基于樹的方法** :決策樹和隨機(jī)森林是用于這個(gè)目的的流行的基于樹的方法。在這些方法中,基于最重要的特征來預(yù)測(cè)目標(biāo)變量創(chuàng)建了一個(gè)樹結(jié)構(gòu)。每個(gè)特征的重要性是通過基于該特征拆分?jǐn)?shù)據(jù)導(dǎo)致的不純度減少來計(jì)算的。
在決策樹中,選擇信息增益最高的特征作為根節(jié)點(diǎn),并基于該特征拆分?jǐn)?shù)據(jù)。這個(gè)過程遞歸重復(fù),直到滿足停止標(biāo)準(zhǔn),例如最大樹深度或每個(gè)葉子節(jié)點(diǎn)的最小樣本數(shù)。
在隨機(jī)森林中,使用特征和數(shù)據(jù)的隨機(jī)子集來建立多個(gè)決策樹。每個(gè)特征的重要性是通過在所有樹中平均減少不純度來計(jì)算的。這有助于降低模型的方差并提高其可推廣性。
from sklearn.ensemble import RandomForestRegressor
# Load the data
X, y = load_data()
# Create a random forest regressor
rf = RandomForestRegressor(n_estimators=100, random_state=42)
# Fit the model
rf.fit(X, y)
# Get feature importances
importances = rf.feature_importances_
# Print feature importances
for feature, importance in zip(X.columns, importances):
print(feature, importance)
基于樹的方法也可以用于特征提取。在這種情況下,我們可以基于樹的決策邊界提取新的特征。例如,我們可以使用決策樹的葉節(jié)點(diǎn)作為新的二元特征,指示數(shù)據(jù)點(diǎn)是否落在特征空間的該區(qū)域內(nèi)。
** 6.包裝方法** :這是一種特征選擇方法,其中模型在不同的特征子集上進(jìn)行訓(xùn)練和評(píng)估。對(duì)于每個(gè)特征子集,模型的性能進(jìn)行測(cè)量,并選擇基于模型性能的最佳特征子集。
下面是一個(gè)使用遞歸特征消除(RFE)和支持向量機(jī)(SVM)分類器在 scikit-learn 中實(shí)現(xiàn)包裝方法的示例:
from sklearn.svm import SVC
from sklearn.feature_selection import RFE
from sklearn.datasets import load_iris
# load the iris dataset
data = load_iris()
X = data.data
y = data.target
# create an SVM classifier
svm = SVC(kernel='linear')
# create a feature selector using RFE with SVM
selector = RFE(svm, n_features_to_select=2)
# fit the selector to the data
selector.fit(X, y)
# print the selected features
print(selector.support_)
print(selector.ranking_)
在這個(gè)例子中,我們首先加載鳶尾花數(shù)據(jù)集,并將其分為特征(X)和目標(biāo)(y)。然后我們使用線性核創(chuàng)建一個(gè) SVM 分類器。然后,我們使用 RFE 和 SVM 創(chuàng)建一個(gè)特征選擇器,并將其擬合到數(shù)據(jù)。最后,我們使用選擇器的 support_ 和 ranking_ 屬性打印所選特征。
** 前向選擇:** 前向選擇是一種包裝方法,它涉及迭代地將一個(gè)特征添加到模型中,直到模型的性能停止提高。以下是它在 Python 中的工作方式:
from sklearn.feature_selection import SequentialFeatureSelector
from sklearn.linear_model import LinearRegression
# Load the dataset
X, y = load_dataset()
# Initialize the feature selector
selector = SequentialFeatureSelector(LinearRegression(), n_features_to_select=5, direction='forward')
# Fit the feature selector
selector.fit(X, y)
# Print the selected features
print(selector.support_)
在上面的代碼中,我們首先加載數(shù)據(jù)集,然后使用線性回歸模型和一個(gè)指定要選擇的特征數(shù)量的參數(shù) n_features_to_select 來初始化 SequentialFeatureSelector 對(duì)象。然后,我們?cè)跀?shù)據(jù)集上擬合選擇器并打印所選特征。
后向消除: 后向消除是一種包裝方法,它涉及迭代地將一個(gè)特征從模型中逐步刪除,直到模型的性能停止提高。以下是它在 Python 中的工作方式:
from sklearn.feature_selection import SequentialFeatureSelector
from sklearn.linear_model import LinearRegression
# Load the dataset
X, y = load_dataset()
# Initialize the feature selector
selector = SequentialFeatureSelector(LinearRegression(), n_features_to_select=5, direction='backward')
# Fit the feature selector
selector.fit(X, y)
# Print the selected features
print(selector.support_)
在上面的代碼中,我們使用線性回歸模型和一個(gè)參數(shù) direction='backward' 來初始化 SequentialFeatureSelector 對(duì)象,以執(zhí)行后向消除。然后,我們?cè)跀?shù)據(jù)集上擬合選擇器并打印所選特征。
窮盡搜索: 窮盡搜索是一種過濾方法,它涉及評(píng)估所有可能的特征子集,并根據(jù)評(píng)分標(biāo)準(zhǔn)選擇最佳的特征子集。以下是它在 Python 中的工作方式:
from itertools import combinations
from sklearn.metrics import r2_score
from sklearn.linear_model import LinearRegression
# Load the dataset
X, y = load_dataset()
# Initialize variables
best_score = -float('inf')
best_features = None
# Loop over all possible subsets of features
for k in range(1, len(X.columns) + 1):
for subset in combinations(X.columns, k):
# Train a linear regression model
X_subset = X[list(subset)]
model = LinearRegression().fit(X_subset, y)
# Compute the R2 score
score = r2_score(y, model.predict(X_subset))
# Update the best subset of features
if score > best_score:
best_score = score
best_features = subset
# Print the best subset of features
print(best_features)
在上面的代碼中,我們首先加載數(shù)據(jù)集,然后使用 itertools.combinations 函數(shù)循環(huán)遍歷所有可能的特征子集。對(duì)于每個(gè)子集,我們訓(xùn)練一個(gè)線性回歸模型并計(jì)算 R2 分?jǐn)?shù)。然后,根據(jù)最高的 R2 分?jǐn)?shù)更新最佳特征子集,并打印所選特征。
** 7.嵌入方法** :這些方法涉及在模型訓(xùn)練過程中選擇特征。例如,Lasso 回歸和 Ridge 回歸會(huì)向損失函數(shù)添加懲罰項(xiàng)以鼓勵(lì)稀疏特征選擇。
Lasso 回歸 : Lasso 回歸也會(huì)向損失函數(shù)添加懲罰項(xiàng),但它使用的是模型系數(shù)的絕對(duì)值而不是平方。這導(dǎo)致了一種更加激進(jìn)的特征選擇過程,因?yàn)橐恍┫禂?shù)可以被設(shè)置為精確的零。Lasso 回歸在處理高維數(shù)據(jù)時(shí)特別有用,因?yàn)樗梢杂行У販p少模型使用的特征數(shù)量。
from sklearn.linear_model import Lasso
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
data = load_boston()
X = data.data
y = data.target
# Standardize the features
scaler = StandardScaler()
X = scaler.fit_transform(X)
# Fit the Lasso model
lasso = Lasso(alpha=0.1)
lasso.fit(X, y)
# Get the coefficients
coefficients = lasso.coef_
Ridge 回歸:Ridge 回歸向損失函數(shù)添加懲罰項(xiàng),這鼓勵(lì)模型選擇一組更重要的特征來預(yù)測(cè)目標(biāo)變量。懲罰項(xiàng)與模型系數(shù)的大小的平方成正比,因此它傾向于將系數(shù)縮小到零,而不是將它們精確地設(shè)置為零。
from sklearn.linear_model import Ridge
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
data = load_boston()
X = data.data
y = data.target
# Standardize the features
scaler = StandardScaler()
X = scaler.fit_transform(X)
# Fit the Ridge model
ridge = Ridge(alpha=0.1)
ridge.fit(X, y)
# Get the coefficients
coefficients = ridge.coef_
在這兩種情況下,正則化參數(shù) alpha 控制懲罰項(xiàng)的強(qiáng)度。alpha 值越高,特征選擇越稀疏。
編碼分類變量
編碼分類變量是特征工程中的一個(gè)關(guān)鍵步驟,它涉及將分類變量轉(zhuǎn)換為機(jī)器學(xué)習(xí)算法可以理解的數(shù)字形式。以下是用于編碼分類變量的一些常見技術(shù):
1.獨(dú)熱編碼:
獨(dú)熱編碼是一種將分類變量轉(zhuǎn)換為一組二進(jìn)制特征的技術(shù),其中每個(gè)特征對(duì)應(yīng)于原始變量中的一個(gè)唯一類別。在這種技術(shù)中,為每個(gè)類別創(chuàng)建一個(gè)新的二進(jìn)制列,如果存在該類別,則將值設(shè)置為1,否則設(shè)置為0。
以下是使用 pandas 庫的示例:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({
'color': ['red', 'blue', 'green', 'red', 'yellow', 'blue']
})
# apply one-hot encoding
one_hot_encoded = pd.get_dummies(df['color'])
print(one_hot_encoded)
2.標(biāo)簽編碼:
標(biāo)簽編碼是一種將原始變量中的每個(gè)類別分配一個(gè)唯一數(shù)字值的技術(shù)。在這種技術(shù)中,每個(gè)類別被賦予一個(gè)數(shù)字標(biāo)簽,其中標(biāo)簽的分配基于變量中類別的順序。
以下是使用 scikit-learn 庫的示例:
from sklearn.preprocessing import LabelEncoder
# create a sample dataframe
df = pd.DataFrame({
'color': ['red', 'blue', 'green', 'red', 'yellow', 'blue']
})
# apply label encoding
label_encoder = LabelEncoder()
df['color_encoded'] = label_encoder.fit_transform(df['color'])
print(df)
Fig.3 — Encoding Data
3.序數(shù)編碼:
序數(shù)編碼是一種根據(jù)類別的順序或排名為原始變量中的每個(gè)類別分配一個(gè)數(shù)字值的技術(shù)。在這種技術(shù)中,類別根據(jù)特定標(biāo)準(zhǔn)排序,然后根據(jù)它們?cè)谂判蛑械奈恢梅峙鋽?shù)字值。
以下是使用 category_encoders 庫的示例:
import category_encoders as ce
# create a sample dataframe
df = pd.DataFrame({
'size': ['S', 'M', 'L', 'XL', 'M', 'S']
})
# apply ordinal encoding
ordinal_encoder = ce.OrdinalEncoder(cols=['size'], order=['S', 'M', 'L', 'XL'])
df = ordinal_encoder.fit_transform(df)
print(df)
-
編碼
+關(guān)注
關(guān)注
6文章
942瀏覽量
54831 -
機(jī)器學(xué)習(xí)
+關(guān)注
關(guān)注
66文章
8418瀏覽量
132646 -
預(yù)處理
+關(guān)注
關(guān)注
0文章
33瀏覽量
10485
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
機(jī)器學(xué)習(xí)算法的特征工程與意義詳解
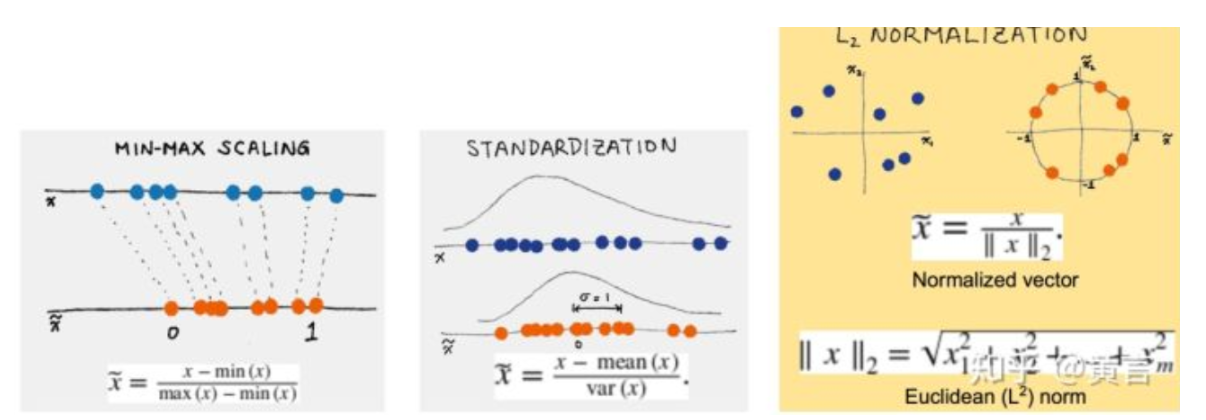
機(jī)器算法學(xué)習(xí)比較
有感FOC算法學(xué)習(xí)與實(shí)現(xiàn)總結(jié)
機(jī)器學(xué)習(xí)特征工程的五個(gè)方面優(yōu)點(diǎn)
SVPWM的原理與算法學(xué)習(xí)課件免費(fèi)下載
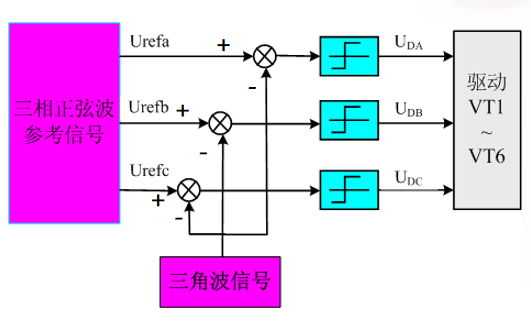
機(jī)器學(xué)習(xí)算法學(xué)習(xí)之特征工程2
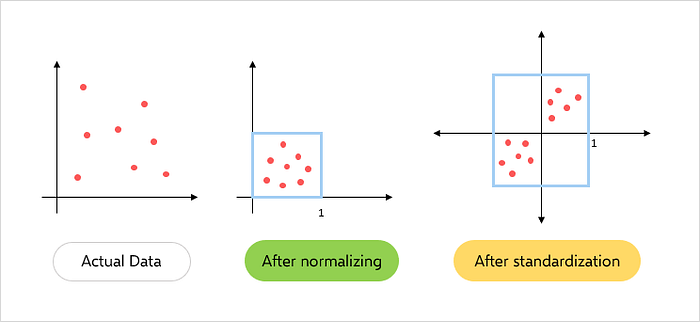
機(jī)器學(xué)習(xí)算法學(xué)習(xí)之特征工程3
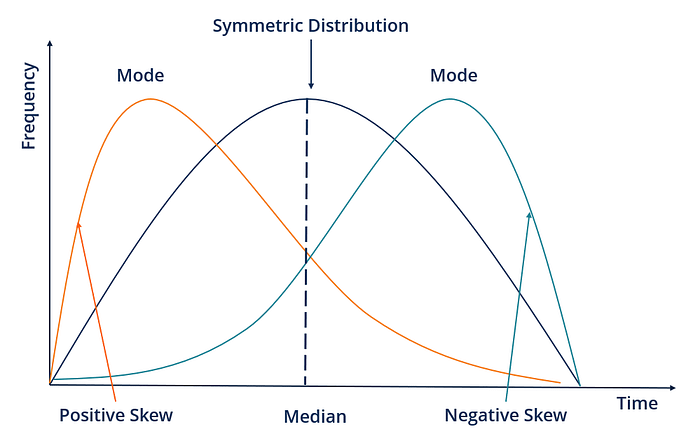
機(jī)器學(xué)習(xí)的經(jīng)典算法與應(yīng)用
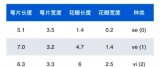
評(píng)論