資料介紹
描述
介紹
大約一年前,我們買了一個 Echo,開始用 Wemo 開關和插頭來裝備我們的房子。能夠告訴 Alexa 從溫暖的床上關掉燈真是太好了。這就是我們 3 歲的女兒出現(xiàn)的地方。太短了,無法到達電燈開關,并且缺乏指導 Alexa 的白話,但有足夠的決心繼續(xù)嘗試。那時我意識到,讓我們的生活變得像成年人一樣方便的相同技術可以讓我們的孩子使用相同的設備。同時,我們可以建立一個與他們一起成長的伙伴。一個可以每周用不同的游戲進行編程的玩具,也可以演變成學習伙伴。最終,她有一天會成為一位朋友,自己學習編程,不僅可以彌合兒童和智能家居之間的鴻溝,
這是Seeed Grove 入門套件的理想選擇。它配備了大量有趣的 I/O 設備,并有可能添加更多設備。更換新的東西(如距離傳感器)就像插入電纜一樣簡單。
為了驅(qū)動盾牌,我正在使用新的Arduino 101 (加拿大和歐洲的 Genuino)。很像 Uno,但顯著增加了陀螺儀、加速度計和藍牙。
最后,為了從 Arduino 控制 Wemo,我正在使用舊的 Android 手機(S4 Active),使用Evothings Studio ,這是一個基于 Cordova 的移動 IDE,可讓您使用 HTML5 和 JS 編寫應用程序。這將通過藍牙與 101 配對,并通過Maker IFTTT通道控制 Wemo。
材料清單
- 安裝了Evothings Viewer應用程序的支持BLE 的移動設備
- 縫紉機/織物(或使用現(xiàn)有玩具)
軟件
指示
阿杜諾/格羅夫
將 Grove Base Shield 放在 Arduino 上,然后連接隨附的傳感器和輸出以匹配下圖。
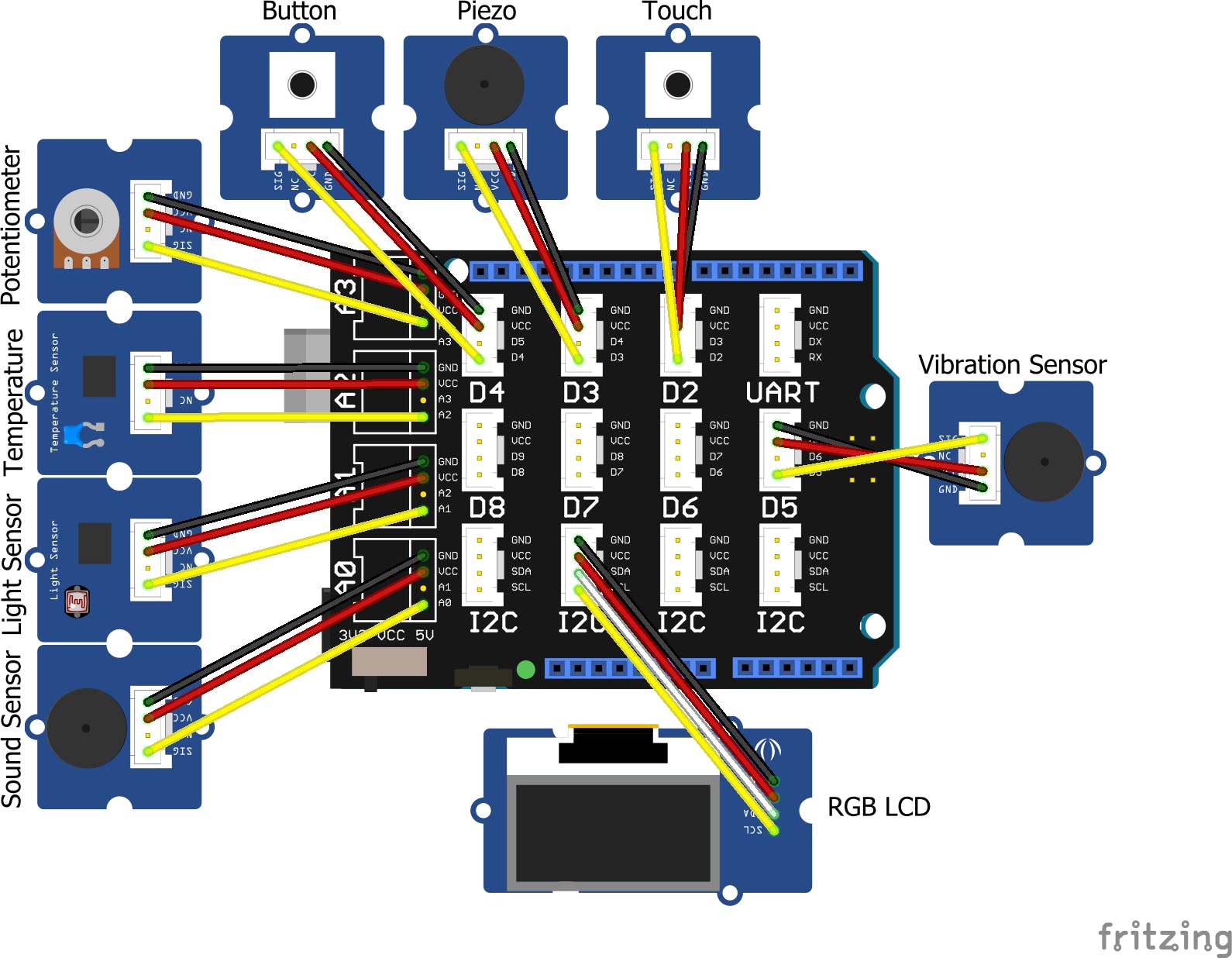
根據(jù)在線說明安裝 Arduino IDE。使用 USB 電纜將軟件運行插入 Arduino 101 板。在 IDE 中,轉(zhuǎn)到 Tools>Board 并選擇“Arduino/Genuino 101”。然后在工具>端口下選擇您的板列出的端口,例如。COM3(Arduino/Genuino 101)。
從GitHub克隆、下載或復制代碼并打開/arduino/Tedduino/Tedduino.ino
/*
* Tedduino.ino
* @author : Justin Revelstoke
* @date : 3/25/2017
* @description : Arduino code for BLE connection and Grove Shield I/O.
* Displays a menu over RGB LCD offering sensor data and
* an example game.
*/
#include
#include
#include "CurieIMU.h"
#include "config.h"
BLEPeripheral blePeripheral;
BLEService bleService(serviceUUID);
BLECharacteristic bleCharacteristic(characteristicUUID, BLERead | BLENotify, 2);
#include "rgb_lcd.h"
rgb_lcd lcd;
const int B = 3975;
const int pinTouch = 2;
const int pinBuzzer = 3;
const int pinButton = 4;
const int pinVibe = 5;
const int pinLED = 13;
const int pinSound = A0;
const int pinLight = A1;
const int pinTemp = A2;
const int pinPot = A3;
int menu = 0;
int previousButtonState = 0;
int length = 15; // the number of notes
char notes[] = "ccggaagffeeddc "; // a space represents a rest
int beats[] = { 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2, 4 };
int tempo = 300;
float aix, aiy, aiz; // accelerometer values
float gix, giy, giz; // gyroscope values
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
lcd.setCursor(0, 0);
pinMode(pinLED, OUTPUT);
pinMode(pinPot, INPUT);
pinMode(pinTouch, INPUT);
pinMode(pinButton, INPUT);
blePeripheral.setLocalName(bleServiceName);
blePeripheral.setAdvertisedServiceUuid(bleService.uuid());
blePeripheral.addAttribute(bleService);
blePeripheral.addAttribute(bleCharacteristic);
const unsigned char bleCharArray[2] = { 0, (char)1 };
bleCharacteristic.setValue(bleCharArray, 2);
blePeripheral.begin();
CurieIMU.begin();
CurieIMU.setGyroRate(25);
CurieIMU.setAccelerometerRate(25);
CurieIMU.setAccelerometerRange(2);
CurieIMU.setGyroRange(250);
}
void loop() {
printGyroAccel();
digitalWrite(pinLED, LOW);
breath(REG_RED);
breath(REG_GREEN);
breath(REG_BLUE);
BLECentral central = blePeripheral.central();
if (central) {
Serial.print("Connected to central: ");
Serial.println(central.address());
while (central.connected()) {
displayMenu();
delay(100);
}
}
delay(100);
}
void nextMenu() {
if (menu == 5) {
menu = 0;
}
else {
menu++;
}
}
void displayMenu() {
resetLCD();
int buttonState = digitalRead(pinButton);
int touchState = digitalRead(pinTouch);
buttonState = buttonState | touchState;
if (previousButtonState != buttonState) {
if (buttonState == 1) {
const unsigned char bleCharArray[2] = { 0, (char)1 };
bleCharacteristic.setValue(bleCharArray, 2);
nextMenu();
delay(1000);
}
else {
const unsigned char bleCharArray[2] = { 0, (char)0 };
bleCharacteristic.setValue(bleCharArray, 2);
}
}
delay(100);
previousButtonState = buttonState;
if (menu == 0) {
printSound();
}
else if (menu == 1) {
printLight();
}
else if (menu == 2) {
printTemp();
}
else if (menu == 3) {
printVibe();
}
else if (menu == 4) {
printPot();
}
else if (menu == 5) {
nightMode();
}
else if (menu == 6) {
printGyroAccel();
}
else {
lcd.print("Error");
}
}
void resetLCD() {
lcd.clear();
lcd.setCursor(0, 0);
}
void printGyroAccel() {
CurieIMU.readAccelerometerScaled(aix, aiy, aiz);
CurieIMU.readGyroScaled(gix, giy, giz);
//CurieIMU.readMotionSensor(aix, aiy, aiz, gix, giy, giz);
lcd.print(aix);
lcd.print(' ');
lcd.print(aiy);
lcd.print(' ');
lcd.print(aiz);
lcd.setCursor(0, 1);
lcd.print(gix);
lcd.print(' ');
lcd.print(giy);
lcd.print(' ');
lcd.print(giz);
delay(100);
resetLCD();
}
int getSound() {
int sensorValue = analogRead(pinSound);
return sensorValue;
}
void printSound() {
int sensorValue = getSound();
lcd.print("Sound:");
if (sensorValue > 0) {
for (int i = 0; i < round(sensorValue/100); i++) {
lcd.print(">");
}
}
}
int getLight() {
int sensorValue = analogRead(pinLight);
return sensorValue;
}
void printLight() {
// range 0 - 1023
int sensorValue = getLight();
lcd.print("Light:");
if (sensorValue > 0) {
for (int i = 0; i < round(sensorValue/100); i++) {
lcd.print(">");
}
}
}
int getTemp() {
int sensorValue = analogRead(pinTemp);
float resistance = (float)(1023-sensorValue)*10000/sensorValue;
int temperature = 1/(log(resistance/10000)/B+1/298.15)-273.15;
return temperature;
}
void printTemp() {
lcd.print("Temp:");
lcd.print(getTemp());
lcd.print("F");
}
int getPot() {
return analogRead(pinPot);
}
void printPot() {
lcd.print("Pot:");
lcd.print(getPot());
}
int getVibe() {
return digitalRead(pinVibe);
}
void printVibe() {
lcd.print("Vibe:");
lcd.print(getVibe());
}
void nightMode() {
bool warm = false;
bool dark = false;
bool quiet = false;
if (getTemp() >= 50) {
lcd.print("Warm ");
warm = true;
}
else {
lcd.print("Cold ");
}
if (getLight() == 0) {
lcd.print("Dark ");
dark = true;
}
else {
lcd.print("Light ");
}
if (getSound() < 100) {
lcd.print("Quiet ");
quiet = true;
}
else {
lcd.print("Loud ");
}
if (warm && dark && quiet) {
playSong();
}
}
void playSong() {
for (int i = 0; i < length; i++) {
if (notes[i] == ' ') {
delay(beats[i] * tempo); // rest
}
else {
playNote(notes[i], beats[i] * tempo);
}
// pause between notes
delay(tempo / 2);
}
}
void playTone(int tone, int duration) {
for (long i = 0; i < duration * 1000L; i += tone * 2) {
digitalWrite(pinBuzzer, HIGH);
delayMicroseconds(tone);
digitalWrite(pinBuzzer, LOW);
delayMicroseconds(tone);
}
}
void playNote(char note, int duration) {
char names[] = { 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' };
int tones[] = { 1915, 1700, 1519, 1432, 1275, 1136, 1014, 956 };
// play the tone corresponding to the note name
for (int i = 0; i < 8; i++) {
if (names[i] == note) {
playTone(tones[i], duration);
}
}
}
void breath(unsigned char color)
{
for(int i=0; i<255; i++)
{
lcd.setPWM(color, i);
delay(5);
}
delay(500);
for(int i=254; i>=0; i--)
{
lcd.setPWM(color, i);
delay(5);
}
delay(500);
}
確保config.h在同一目錄中
/*
* config.h
* @author : Justin Revelstoke
* @date : 3/25/2017
* @description : Configuration
*/
// You're device will appear in bluetooth connection lists under this name
#define bleServiceName "Tedduino"
#define serviceUUID "180D"
#define characteristicUUID "2A37"
單擊 Arduino IDE 中的上傳按鈕,控制臺應顯示:
開始下載腳本...
SUCCESS:草圖將在大約 5 秒內(nèi)執(zhí)行。
如果它工作正常,您現(xiàn)在應該看到 LCD 呈紅色、綠色和藍色閃爍。
此時 Arduino 正在宣傳 BLE 連接,但具有許多“離線”功能。按下按鈕或觸摸傳感器將在 LCD 上的各種顯示中旋轉(zhuǎn),顯示連接傳感器的讀數(shù)。還有一個示例“游戲”,要求您將 Arduino 放置在明亮|黑暗、溫暖|寒冷或安靜|響亮的地方。成功后會播放一??首歌:)
進化論
現(xiàn)在設置 BLE 配套應用程序。如果您有興趣了解有關低功耗藍牙的更多信息,我強烈推薦Adafruit的這本入門書。此外,如果您想使用 BLE 連接而不需要發(fā)出 Web 請求,Blynk是快速連接到 Arduino 的好方法。它只是不支持我在 BLE 上需要的功能。
因此,我們需要做的第一件事是下載并安裝Evothings Studio (撰寫本文時版本為 2.2.0)。請務必從下載頁面獲取云令牌。
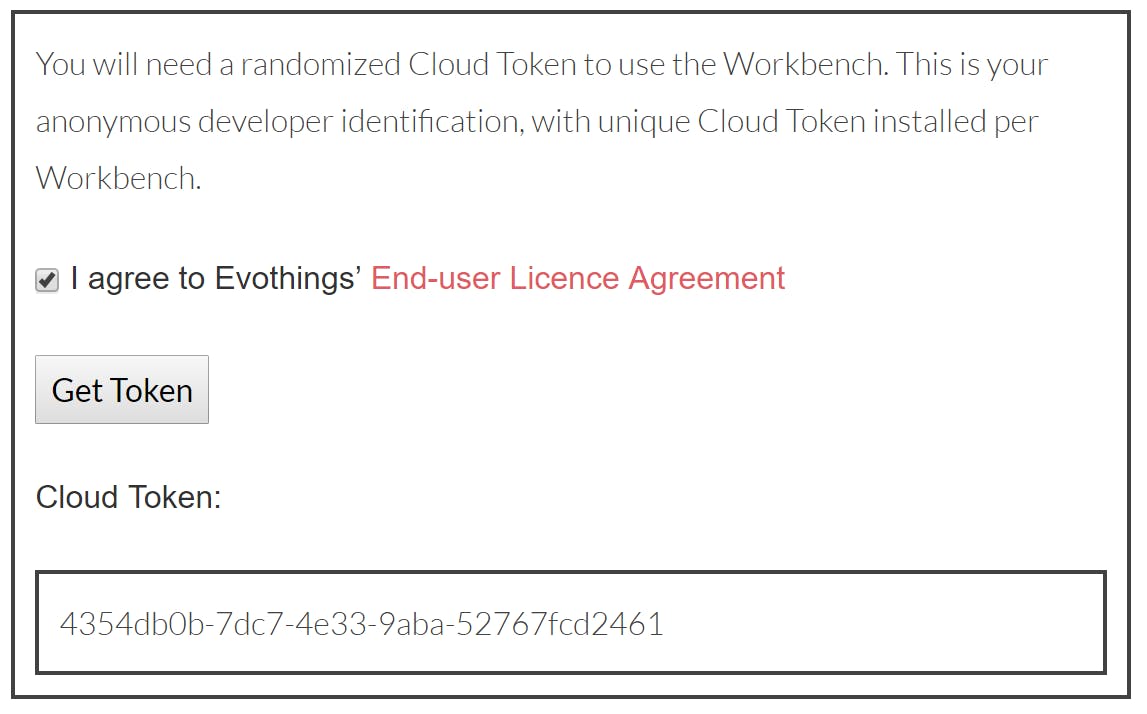
接下來,從應用商店下載 Evothings Viewer 。
將 Studio 中的連接密鑰輸入到查看器中以鏈接它們。

現(xiàn)在,從您之前克隆的同一個存儲庫中打開cordova文件夾并找到evothings.json文件。單擊該文件并將其拖入 Evothings Studio 以創(chuàng)建項目。你現(xiàn)在應該看到...

如果您單擊“運行”,該應用程序應在您的手機上啟動
IFTTT 集成
為了完成 Evothings 代碼,我們需要在 IFTTT 中設置一個 Maker 頻道。為此,請訪問IFTTT 。如果您還沒有帳戶,請登錄或創(chuàng)建一個帳戶。然后在“我的小程序”下單擊“新建小程序”。按照下面的一系列圖片設置您的 Maker 頻道。請務必在設置您的網(wǎng)址后復制 Maker 為您生成的密鑰。科爾多瓦需要它。創(chuàng)建兩個通道,一個使用“tedduino_on”作為觸發(fā)器,另一個使用“tedduino_off”。相應地為 WeMo 操作選擇“打開”和“關閉”。
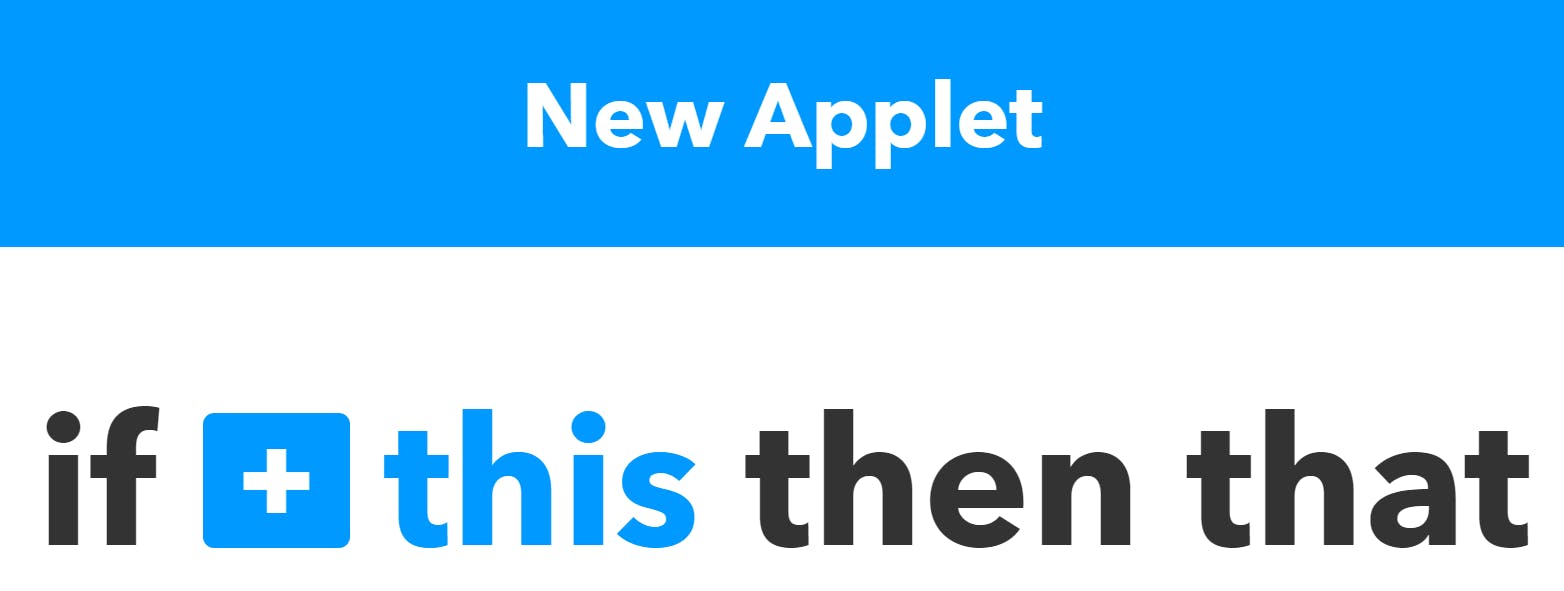
如果一切順利,我們現(xiàn)在應該有兩個通道和一個 Maker 密鑰。您可以在瀏覽器中測試 URL(它們應該類似于)。
把它們放在一起
從GitHub打開app文件夾中的index.html回到 cordova 代碼。找到這一行:
var
makerKey
= '/with/key/INSERT YOUR KEY HERE';
并用您的制造商頻道密鑰替換“在此處插入您的密鑰”。保存并將您的 Evothings 工作室重新連接到查看器。
恭喜!此時您應該能夠運行 Evothings 應用程序并單擊“連接”。如果它成功連接到 Arduino,您可以按“測試”,LCD 應該停止改變顏色。您還應該能夠按下 Grove 按鈕來打開和關閉燈。
如果您對縫紉機很方便,我建議您設計自己的娃娃。否則,您可以輕松地將這些部件改裝成現(xiàn)有的部件。只需用 Arduino 電池組替換 USB 電纜即可。熱膠是保持部件到位的好方法。玩得開心!
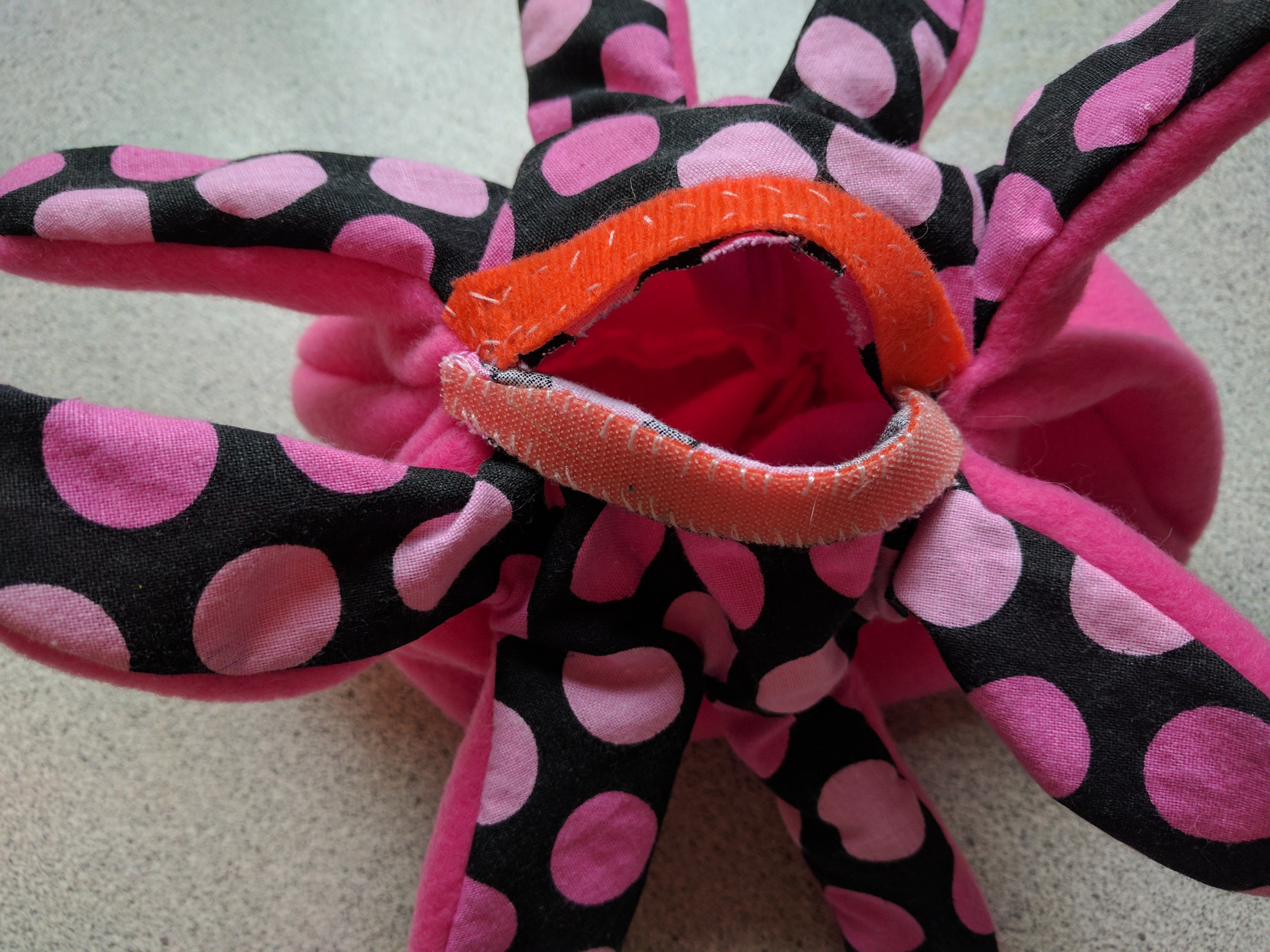
隨著時間的推移,我們家的更多方面變得自動化,我希望制造更多這樣的設備,讓我們的孩子更加獨立。
?
- 音樂播放器小程序特效HTML5源代碼 5次下載
- canvas鴨子射擊小游戲特效HTML5源代碼 6次下載
- 鋼琴應用程序web特效HTML5源代碼 4次下載
- 天天消消樂游戲程序HTML5源代碼 14次下載
- 使用HTML5實現(xiàn)井字棋小游戲的算法和代碼講解
- 解析html工具應用程序免費下載 11次下載
- HTML5應用程序攻擊研究 0次下載
- 基于HTML5實現(xiàn)WebGIS中地理要素的展示與交互 0次下載
- HTML5在汽車領域的應用解析 0次下載
- HTML5移動Web開發(fā)指南 5次下載
- 幾款HTML5游戲引擎一覽 1次下載
- HTML5圖表動畫應用 2次下載
- 基于響應式Web設計的HTML5和CSS3實際案例 6次下載
- HTML5基礎 0次下載
- HTML5實戰(zhàn)_陶國榮 0次下載
- 超好用的開源IP地址管理系統(tǒng),告別傳統(tǒng)Excel統(tǒng)計方式! 5287次閱讀
- 如何使用Tokio 和 Tracing模塊構建異步的網(wǎng)絡應用程序 542次閱讀
- 基于OpenHarmony編寫GPIO平臺驅(qū)動和應用程序 734次閱讀
- 基于RTOS的應用程序的五個最佳實踐技巧 842次閱讀
- PreEmptiveProtection:全面的移動應用程序保護 529次閱讀
- 用中文編寫的博途SCL程序 884次閱讀
- 怎么使用uboot引導應用程序? 1360次閱讀
- Verilog程序編寫規(guī)范 3757次閱讀
- 淺談RAM 執(zhí)行應用程序 3060次閱讀
- 適合移動應用開發(fā)的編程語言有哪些 4455次閱讀
- 單片機的程序編寫 4202次閱讀
- 如何使用HyperledgeFabric網(wǎng)絡react.js來構建Web應用程序 1149次閱讀
- 基于單片機應用程序編寫的七大步驟分享 3997次閱讀
- JavaScript讓HTML靜態(tài)頁面?zhèn)髦档姆椒?/a> 5828次閱讀
- ARDUINO IDE編寫和AVR單片機程序的下載 9769次閱讀
下載排行
本周
- 1山景DSP芯片AP8248A2數(shù)據(jù)手冊
- 1.06 MB | 532次下載 | 免費
- 2RK3399完整板原理圖(支持平板,盒子VR)
- 3.28 MB | 339次下載 | 免費
- 3TC358743XBG評估板參考手冊
- 1.36 MB | 330次下載 | 免費
- 4DFM軟件使用教程
- 0.84 MB | 295次下載 | 免費
- 5元宇宙深度解析—未來的未來-風口還是泡沫
- 6.40 MB | 227次下載 | 免費
- 6迪文DGUS開發(fā)指南
- 31.67 MB | 194次下載 | 免費
- 7元宇宙底層硬件系列報告
- 13.42 MB | 182次下載 | 免費
- 8FP5207XR-G1中文應用手冊
- 1.09 MB | 178次下載 | 免費
本月
- 1OrCAD10.5下載OrCAD10.5中文版軟件
- 0.00 MB | 234315次下載 | 免費
- 2555集成電路應用800例(新編版)
- 0.00 MB | 33566次下載 | 免費
- 3接口電路圖大全
- 未知 | 30323次下載 | 免費
- 4開關電源設計實例指南
- 未知 | 21549次下載 | 免費
- 5電氣工程師手冊免費下載(新編第二版pdf電子書)
- 0.00 MB | 15349次下載 | 免費
- 6數(shù)字電路基礎pdf(下載)
- 未知 | 13750次下載 | 免費
- 7電子制作實例集錦 下載
- 未知 | 8113次下載 | 免費
- 8《LED驅(qū)動電路設計》 溫德爾著
- 0.00 MB | 6656次下載 | 免費
總榜
- 1matlab軟件下載入口
- 未知 | 935054次下載 | 免費
- 2protel99se軟件下載(可英文版轉(zhuǎn)中文版)
- 78.1 MB | 537798次下載 | 免費
- 3MATLAB 7.1 下載 (含軟件介紹)
- 未知 | 420027次下載 | 免費
- 4OrCAD10.5下載OrCAD10.5中文版軟件
- 0.00 MB | 234315次下載 | 免費
- 5Altium DXP2002下載入口
- 未知 | 233046次下載 | 免費
- 6電路仿真軟件multisim 10.0免費下載
- 340992 | 191187次下載 | 免費
- 7十天學會AVR單片機與C語言視頻教程 下載
- 158M | 183279次下載 | 免費
- 8proe5.0野火版下載(中文版免費下載)
- 未知 | 138040次下載 | 免費
評論