python讀取數(shù)據(jù)庫數(shù)據(jù) python查詢數(shù)據(jù)庫 python數(shù)據(jù)庫連接
Python是一門高級編程語言,廣泛應(yīng)用于各種領(lǐng)域。其中,Python在數(shù)據(jù)庫處理方面有著廣泛的應(yīng)用,可以輕松地連接各種數(shù)據(jù)庫,獲取數(shù)據(jù)庫中的數(shù)據(jù),并對數(shù)據(jù)進行增刪改查等操作。本文將詳細介紹Python如何連接數(shù)據(jù)庫、讀取數(shù)據(jù)庫數(shù)據(jù)以及如何進行查詢操作。
一、Python連接數(shù)據(jù)庫
Python連接數(shù)據(jù)庫一般需要使用第三方庫,包括MySQLDB、sqlite3、psycopg2等庫。其中MySQLDB是Python連接MySQL數(shù)據(jù)庫的一個重要庫,sqlite3可以連接SQLite數(shù)據(jù)庫,psycopg2可以連接PostgreSQL數(shù)據(jù)庫。下面將以MySQL為例進行說明。
首先需要安裝MySQLDB庫,可以在Python環(huán)境下直接使用pip進行安裝:
```python
pip install MySQL-python
```
安裝完成后,需要在Python代碼中導(dǎo)入MySQLDB庫。
```python
import MySQLdb
```
在連接數(shù)據(jù)庫之前,需要準備好數(shù)據(jù)庫的相關(guān)信息,包括主機名、用戶名、密碼、數(shù)據(jù)庫名等。接下來,我們使用MySQLdb庫中的`connect()`方法進行連接。下面是一個樣例代碼:
```python
import MySQLdb
host = 'localhost'
user = 'root'
passwd = '123456'
db_name = 'test'
db = MySQLdb.connect(host=host, user=user, passwd=passwd, db=db_name, charset='utf8')
cursor = db.cursor()
```
其中,`host`為數(shù)據(jù)庫主機名,`user`為用戶名,`passwd`為密碼,`db_name`為數(shù)據(jù)庫名。另外,`charset`參數(shù)為設(shè)置連接字符集,一般使用utf8。此處的`cursor()`方法是為了創(chuàng)建一個游標對象,用于執(zhí)行SQL語句。
二、Python讀取數(shù)據(jù)庫數(shù)據(jù)
連接上數(shù)據(jù)庫之后,就可以開始讀取數(shù)據(jù)庫中的數(shù)據(jù)了。Python讀取MySQL數(shù)據(jù)庫中的數(shù)據(jù)可以通過以下幾種方式:
1. 使用`fetchone()`方法獲取一條數(shù)據(jù)。
```python
sql = "select * from students limit 1"
cursor.execute(sql)
result = cursor.fetchone()
print(result)
```
其中,`fetchone()`方法用于獲取查詢結(jié)果集中的第一條記錄,返回一個元組類型。在樣例代碼中,我們查詢了students表中的第一條記錄,并輸出該記錄。
2. 使用`fetchall()`方法獲取所有數(shù)據(jù)。
```python
sql = "select * from students"
cursor.execute(sql)
results = cursor.fetchall()
for result in results:
print(result)
```
`fetchall()`方法用于獲取查詢結(jié)果集中的所有記錄,返回一個元組類型的列表。在樣例代碼中,我們查詢了students表中的所有記錄,并逐條輸出結(jié)果。
3. 使用`fetchmany()`方法獲取指定數(shù)量的數(shù)據(jù)。
```python
sql = "select * from students"
cursor.execute(sql)
results = cursor.fetchmany(10)
for result in results:
print(result)
```
`fetchmany()`方法用于獲取指定數(shù)量的記錄,返回一個元組類型的列表。在樣例代碼中,我們查詢了students表中的所有記錄,并輸出結(jié)果前10條記錄。
三、Python查詢數(shù)據(jù)庫
在Python中,可以使用SQL語句來查詢數(shù)據(jù)庫中的數(shù)據(jù)。SQL語句是一種用于訪問和操作關(guān)系數(shù)據(jù)庫中數(shù)據(jù)的計算機語言,在Python中可以使用`execute()`方法執(zhí)行SQL查詢語句,并使用`fetchxxx()`方法獲取查詢結(jié)果。
下面是一個使用SQL語句查詢MySQL數(shù)據(jù)庫的樣例:
```python
sql = "select * from students where score > 90"
cursor.execute(sql)
results = cursor.fetchall()
for result in results:
print(result)
```
在樣例代碼中,我們使用SQL查詢語句查詢了score大于90的students表中所有記錄,并輸出查詢結(jié)果。
四、Python數(shù)據(jù)庫操作
Python連接數(shù)據(jù)庫之后,可以對數(shù)據(jù)庫進行增刪改查等操作。下面分別介紹這些操作。
1. 插入數(shù)據(jù)
在MySQL中插入數(shù)據(jù)可以使用SQL語句,示例代碼如下:
```python
sql = "insert into students(name, age, score) values('Tom', 20, 95)"
cursor.execute(sql)
db.commit()
```
2. 更新數(shù)據(jù)
使用SQL語句更新數(shù)據(jù),示例代碼如下:
```python
sql = "update students set score=98 where id=1"
cursor.execute(sql)
db.commit()
```
3. 刪除數(shù)據(jù)
使用SQL語句刪除數(shù)據(jù),示例代碼如下:
```python
sql = "delete from students where id=1"
cursor.execute(sql)
db.commit()
```
綜上所述,Python連接數(shù)據(jù)庫、讀取數(shù)據(jù)庫數(shù)據(jù)、查詢數(shù)據(jù)庫和操作數(shù)據(jù)庫都是非常重要的應(yīng)用,這里我們以MySQL為例,介紹了連接MySQL數(shù)據(jù)庫的方法、如何讀取數(shù)據(jù)庫數(shù)據(jù)、查詢數(shù)據(jù)庫和操作數(shù)據(jù)庫,希望對大家有所幫助。
-
SQL
+關(guān)注
關(guān)注
1文章
778瀏覽量
44627 -
數(shù)據(jù)庫
+關(guān)注
關(guān)注
7文章
3874瀏覽量
65402 -
python
+關(guān)注
關(guān)注
56文章
4821瀏覽量
85635
發(fā)布評論請先 登錄
相關(guān)推薦
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)——MongoDB數(shù)據(jù)庫文件拷貝后服務(wù)無法啟動的數(shù)據(jù)恢復(fù)
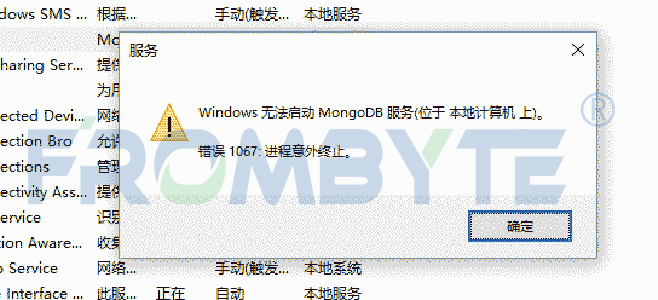
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—SQL Server附加數(shù)據(jù)庫提示“錯誤 823”的數(shù)據(jù)恢復(fù)案例
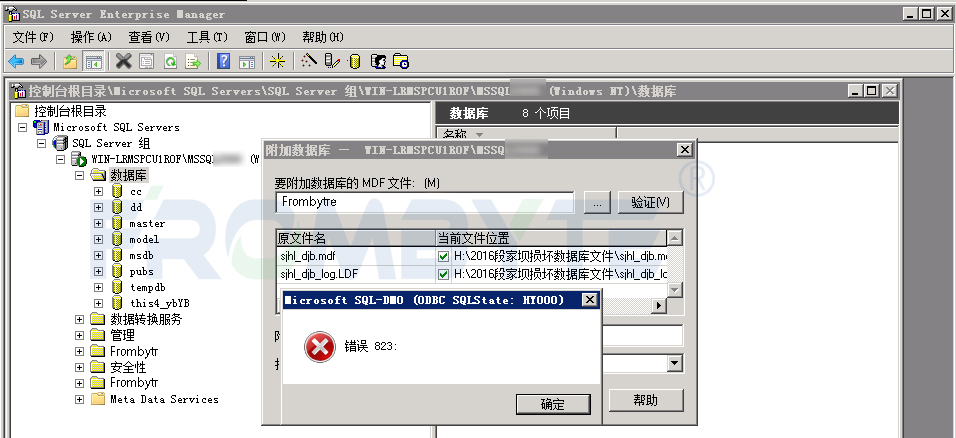
適用于MySQL和MariaDB的Python連接器:可靠的MySQL數(shù)據(jù)連接器和數(shù)據(jù)庫
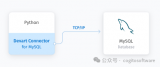
MySQL數(shù)據(jù)庫的安裝
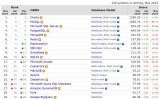
適用于Oracle的Python連接器:可訪問托管以及非托管的數(shù)據(jù)庫
云數(shù)據(jù)庫是哪種數(shù)據(jù)庫類型?
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—Mysql數(shù)據(jù)庫表記錄丟失的數(shù)據(jù)恢復(fù)流程
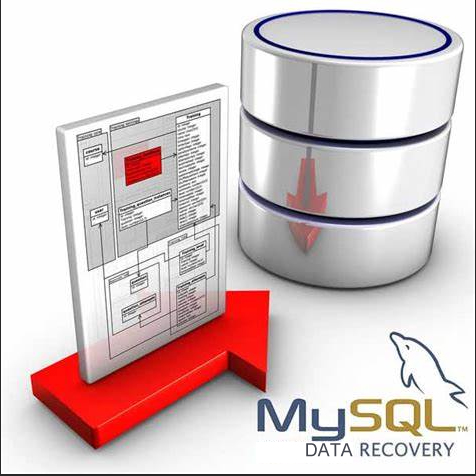
數(shù)據(jù)庫事件觸發(fā)的設(shè)置和應(yīng)用
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—MYSQL數(shù)據(jù)庫ibdata1文件損壞的數(shù)據(jù)恢復(fù)案例
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—通過拼接數(shù)據(jù)庫碎片恢復(fù)SQLserver數(shù)據(jù)庫
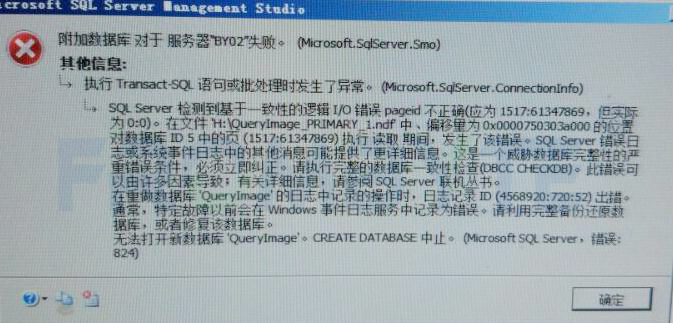
Oracle數(shù)據(jù)恢復(fù)—異常斷電后Oracle數(shù)據(jù)庫啟庫報錯的數(shù)據(jù)恢復(fù)案例
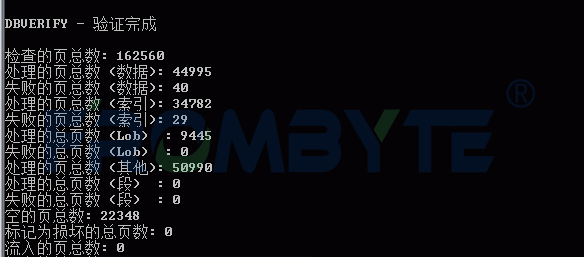
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—SQL Server數(shù)據(jù)庫出現(xiàn)823錯誤的數(shù)據(jù)恢復(fù)案例
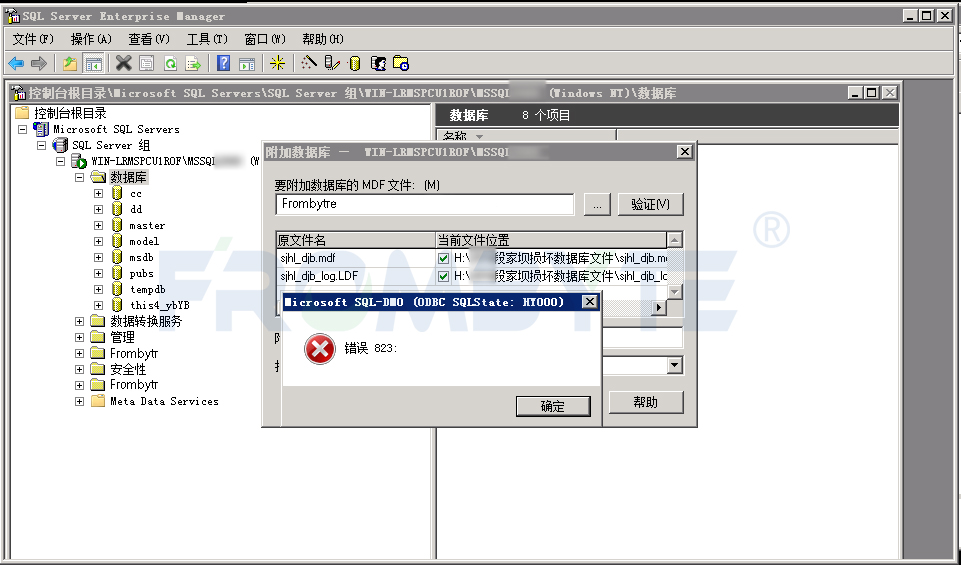
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—SqlServer數(shù)據(jù)庫底層File Record被截斷為0的數(shù)據(jù)恢復(fù)案例
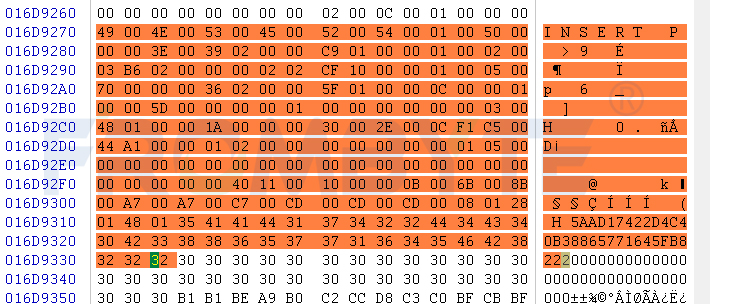
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—SQL Server數(shù)據(jù)庫所在分區(qū)空間不足報錯的數(shù)據(jù)恢復(fù)案例
數(shù)據(jù)庫數(shù)據(jù)恢復(fù)—raid5陣列上層Sql Server數(shù)據(jù)庫數(shù)據(jù)恢復(fù)案例
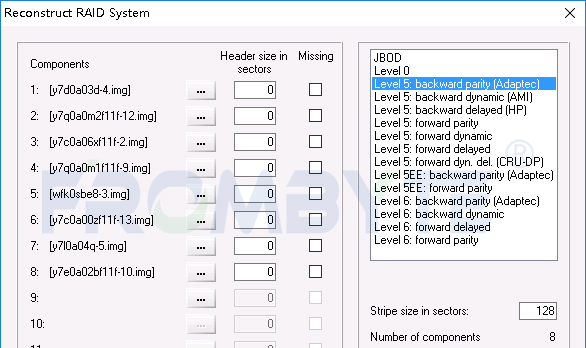
評論