鏈表是由一連串節(jié)點(diǎn)組成的數(shù)據(jù)結(jié)構(gòu),每個節(jié)點(diǎn)包含一個數(shù)據(jù)值和一個指向下一個節(jié)點(diǎn)的指針。鏈表可以在頭部和尾部插入和刪除節(jié)點(diǎn),因此可以在任何地方插入和刪除節(jié)點(diǎn),從而使其變得靈活和易于實(shí)現(xiàn)。
鏈表通常用于實(shí)現(xiàn)有序集合,例如隊(duì)列和雙向鏈表。鏈表的優(yōu)點(diǎn)是可以快速隨機(jī)訪問節(jié)點(diǎn),而缺點(diǎn)是插入和刪除操作相對慢一些,因?yàn)樾枰苿庸?jié)點(diǎn)。此外,鏈表的長度通常受限于內(nèi)存空間,因此當(dāng)鏈表變得很長時,可能需要通過分頁或鏈表分段等方式來管理其內(nèi)存。
下面是一套封裝好的單鏈表框架,包括創(chuàng)建鏈表、插入節(jié)點(diǎn)、刪除節(jié)點(diǎn)、修改節(jié)點(diǎn)、遍歷節(jié)點(diǎn)和清空鏈表等常見操作,其中每個節(jié)點(diǎn)存儲一個結(jié)構(gòu)體變量,該結(jié)構(gòu)體中包含一個名為data的int類型成員。
#include
#include
?
// 鏈表節(jié)點(diǎn)結(jié)構(gòu)體
typedef struct ListNode {
int data; // 節(jié)點(diǎn)數(shù)據(jù)
struct ListNode *next; // 下一個節(jié)點(diǎn)的指針
} ListNode;
?
// 創(chuàng)建一個新節(jié)點(diǎn)
ListNode *createNode(int data) {
ListNode *node = (ListNode*) malloc(sizeof(ListNode));
node->data = data;
node->next = NULL;
return node;
}
?
// 在鏈表頭部插入一個新節(jié)點(diǎn)
ListNode *insertNodeAtHead(ListNode *head, int data) {
ListNode *node = createNode(data);
node->next = head;
return node;
}
?
// 在鏈表尾部插入一個新節(jié)點(diǎn)
ListNode *insertNodeAtTail(ListNode *head, int data) {
ListNode *node = createNode(data);
if(head == NULL) {
return node;
} else {
ListNode *current = head;
while(current->next != NULL) {
current = current->next;
}
current->next = node;
return head;
}
}
?
// 刪除鏈表中第一個值為data的節(jié)點(diǎn)
ListNode *deleteNode(ListNode *head, int data) {
if(head == NULL) {
return NULL;
}
if(head->data == data) {
ListNode *current = head;
head = head->next;
free(current);
return head;
}
ListNode *current = head;
while(current->next != NULL && current->next->data != data) {
current = current->next;
}
if(current->next != NULL) {
ListNode *deleteNode = current->next;
current->next = deleteNode->next;
free(deleteNode);
}
return head;
}
?
// 修改鏈表中第一個值為oldData的節(jié)點(diǎn)的數(shù)據(jù)為newData
void updateNode(ListNode *head, int oldData, int newData) {
ListNode *current = head;
while(current != NULL) {
if(current->data == oldData) {
current->data = newData;
break;
} else {
current = current->next;
}
}
}
?
// 遍歷鏈表
void traverseList(ListNode *head) {
ListNode *current = head;
while(current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("
");
}
?
// 清空鏈表,釋放所有節(jié)點(diǎn)的內(nèi)存空間
void clearList(ListNode *head) {
while(head != NULL) {
ListNode *current = head;
head = head->next;
free(current);
}
}
?
// 示例程序
int main() {
ListNode *head = NULL;
head = insertNodeAtHead(head, 1);
head = insertNodeAtHead(head, 2);
head = insertNodeAtTail(head, 3);
traverseList(head);
head = deleteNode(head, 2);
traverseList(head);
updateNode(head, 1, 4);
traverseList(head);
clearList(head);
return 0;
}
在上述代碼中,定義了一個節(jié)點(diǎn)結(jié)構(gòu)體ListNode,其中包含一個int類型的data成員和一個指向下一個節(jié)點(diǎn)的指針。接著定義了用于創(chuàng)建新節(jié)點(diǎn)、插入節(jié)點(diǎn)、刪除節(jié)點(diǎn)、修改節(jié)點(diǎn)、遍歷節(jié)點(diǎn)和清空鏈表等操作的子函數(shù),并在main函數(shù)中演示了這些操作的使用例子。在使用完鏈表后一定要調(diào)用clearList函數(shù)釋放內(nèi)存空間。
審核編輯:湯梓紅
-
內(nèi)存
+關(guān)注
關(guān)注
8文章
3025瀏覽量
74047 -
C語言
+關(guān)注
關(guān)注
180文章
7604瀏覽量
136824 -
函數(shù)
+關(guān)注
關(guān)注
3文章
4331瀏覽量
62618 -
指針
+關(guān)注
關(guān)注
1文章
480瀏覽量
70563 -
數(shù)據(jù)結(jié)構(gòu)
+關(guān)注
關(guān)注
3文章
573瀏覽量
40130
發(fā)布評論請先 登錄
相關(guān)推薦
Qt(C++)使用SQLite數(shù)據(jù)庫完成數(shù)據(jù)增刪改查
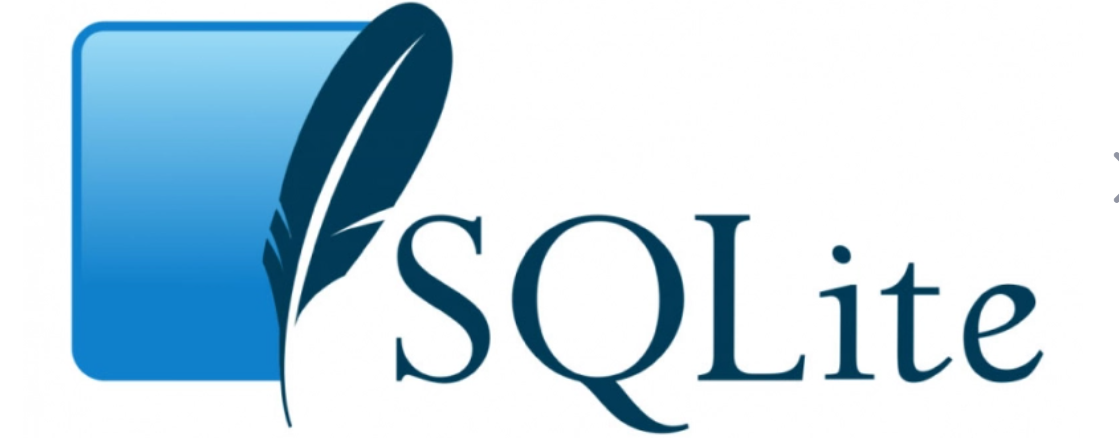
Mybatis自動生成增刪改查代碼
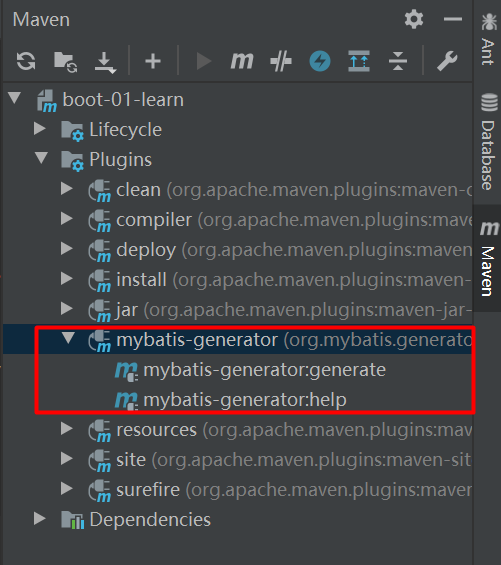
Qt(C++)使用SQLite數(shù)據(jù)庫完成數(shù)據(jù)增刪改查
如何用php調(diào)用mysql數(shù)據(jù)庫實(shí)現(xiàn)增刪改查
python是如何實(shí)現(xiàn)hbase增刪改查的
C語言實(shí)現(xiàn)單鏈表舉例
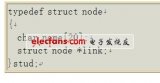
評論